(You can use it to Create your own Calculator and may be which is more efficient than mine..Just in case u find any flaws in this, pls do mail me abt it..)
Screenshot of "Calculator" that I created in Java
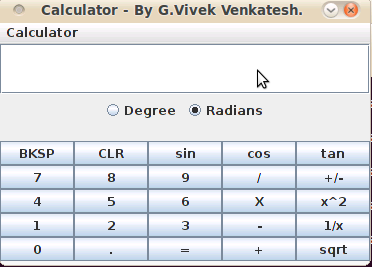
Download the "Calculator"
Download the Source Code
Code of Calculator.java
// Initial Declarations
import javax.swing.*;
import javax.swing.event.*;
import java.awt.*;
import java.awt.event.*;
// Creating a class named Calculator
class Calculator
{
// Components that are required to create the Calculator
JFrame frame = new JFrame();
// Creating the Menu Bar
JMenuBar menubar = new JMenuBar();
//---> Creating the "Calculator-->Exit" Menu
JMenu firstmenu = new JMenu("Calculator");
JMenuItem exitmenu = new JMenuItem("Exit");
// Creating The TextArea that gets the value
JTextField editor = new JTextField();
JRadioButton degree = new JRadioButton("Degree");
JRadioButton radians = new JRadioButton("Radians");
String[] buttons = {"BKSP","CLR","sin","cos","tan","7","8","9","/","+/-","4","5","6","X","x^2","1","2","3","-","1/x","0",".","=","+","sqrt"};
JButton[] jbuttons = new JButton[26];
double buf=0,result;
boolean opclicked=false,firsttime=true;
String last_op;
// Creating a Constructor to Initialize the Calculator Window
public Calculator()
{
frame.setSize(372,270);
frame.setTitle("Calculator - By G.Vivek Venkatesh.");
frame.setLayout(new BorderLayout());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
ButtonHandler bhandler = new ButtonHandler();
menubar.add(firstmenu);
firstmenu.add(exitmenu);
exitmenu.setActionCommand("mExit");
exitmenu.addActionListener(bhandler);
editor.setPreferredSize(new Dimension(20,50));
Container buttoncontainer = new Container();
buttoncontainer.setLayout(new GridLayout(5,5));
for(int i=0;i
jbuttons[i] = new JButton(buttons[i]);
jbuttons[i].setActionCommand(buttons[i]);
jbuttons[i].addActionListener(bhandler);
buttoncontainer.add(jbuttons[i]);
}
JPanel degrad = new JPanel();
degrad.setLayout(new FlowLayout());
ButtonGroup bg1 = new ButtonGroup();
bg1.add(degree);
bg1.add(radians);
degrad.add(degree);
radians.setSelected(true);
degrad.add(radians);
frame.setJMenuBar(menubar);
frame.add(editor,BorderLayout.NORTH);
frame.add(degrad,BorderLayout.CENTER);
frame.add(buttoncontainer,BorderLayout.SOUTH);
frame.setVisible(true);
}
// Class that handles the Events (that implements ActionListener)
public class ButtonHandler implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
String action = e.getActionCommand();
if(action == "0" || action=="1" || action=="2" || action=="3" || action=="4" || action=="5" || action=="6" || action=="7" || action=="8" || action=="9" || action==".")
{
if(opclicked == false)
editor.setText(editor.getText() + action);
else
{
editor.setText(action);
opclicked = false;
}
}
if(action == "CLR")
{
editor.setText("");
buf=0;
result=0;
opclicked=false;
firsttime=true;
last_op=null;
}
//Addition
if(action=="+")
{
firsttime = false;
if(last_op!="=" && last_op!="sqrt" && last_op!="1/x" && last_op!="x^2" && last_op!="+/-")
{
buf = buf + Double.parseDouble(editor.getText());
editor.setText(Double.toString(buf));
last_op = "+";
opclicked=true;
}
else
{
opclicked=true;
last_op = "+";
}
}
// Subtraction
if(action=="-")
{
if(firsttime==true)
{
buf = Double.parseDouble(editor.getText());
firsttime = false;
opclicked=true;
last_op = "-";
}
else
{
if(last_op!="=" && last_op!="sqrt" && last_op!="1/x" && last_op!="x^2" && last_op!="+/-")
{
buf = buf - Double.parseDouble(editor.getText());
editor.setText(Double.toString(buf));
last_op = "-";
opclicked=true;
}
else
{
opclicked=true;
last_op = "-";
}
}
}
//Multiplication
if(action=="X")
{
if(firsttime==true)
{
buf = Double.parseDouble(editor.getText());
firsttime = false;
opclicked = true;
last_op = "X";
}
else
{
if(last_op!="=" && last_op!="sqrt" && last_op!="1/x" && last_op!="x^2" && last_op!="+/-")
{
buf = buf * Double.parseDouble(editor.getText());
editor.setText(Double.toString(buf));
last_op = "X";
opclicked=true;
}
else
{
opclicked=true;
last_op = "X";
}
}
}
//Division
if(action=="/")
{
if(firsttime==true)
{
buf = Double.parseDouble(editor.getText());
firsttime = false;
opclicked=true;
last_op = "/";
}
else
{
if(last_op!="=" && last_op!="sqrt" && last_op!="1/x" && last_op!="x^2" && last_op!="+/-")
{
buf = buf / Double.parseDouble(editor.getText());
editor.setText(Double.toString(buf));
last_op = "/";
opclicked=true;
}
else
{
opclicked=true;
last_op = "/";
}
}
}
// Equal to
if(action=="=")
{
result = buf;
if(last_op=="+")
{
result = buf + Double.parseDouble(editor.getText());
buf = result;
}
if(last_op=="-")
{
result = buf - Double.parseDouble(editor.getText());
buf = result;
}
if(last_op=="X")
{
result = buf * Double.parseDouble(editor.getText());
buf = result;
}
if(last_op=="/")
{
try
{
result = buf / Double.parseDouble(editor.getText());
}
catch(Exception ex)
{
editor.setText("Math Error " + ex.toString());
}
buf = result;
}
editor.setText(Double.toString(result));
last_op = "=";
}
// Sqrt
if(action=="sqrt")
{
if(firsttime==false)
{
buf = Math.sqrt(buf);
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "sqrt";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = Math.sqrt(buf);
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "sqrt";
}
}
}
// Reciprocal
if(action=="1/x")
{
if(firsttime==false)
{
buf = 1/ buf;
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "1/x";
}
else
{
if(editor.getText()==null)
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = 1 / buf;
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "1/x";
}
}
}
// Square
if(action=="x^2")
{
if(firsttime==false)
{
buf = buf * buf;
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "x^2";
}
else
{
if(editor.getText()==null)
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = buf * buf;
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "x^2";
}
}
}
// Negation +/-
if(action=="+/-")
{
if(firsttime==false)
{
buf = -(buf);
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "+/-";
}
else
{
if(editor.getText()==null)
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = -(buf);
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "+/-";
}
}
}
// Exit
if(action=="mExit")
{
frame.dispose();
System.exit(0);
}
if(action=="mCut")
editor.cut();
if(action=="mCopy")
editor.copy();
if(action=="mPaste")
editor.paste();
if(action=="sin")
{
if(radians.isSelected())
{
if(firsttime==false)
{
buf = Math.sin(Double.parseDouble(editor.getText()));
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "sin";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = Math.sin(Double.parseDouble(editor.getText()));
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "sin";
}
}
}
else
{
if(firsttime==false)
{
double rad=Math.toRadians(Double.parseDouble(editor.getText()));
buf = Math.sin(rad);
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "sin";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
double rad=Math.toRadians(Double.parseDouble(editor.getText()));
buf = Math.sin(rad);
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "sin";
}
}
}
}// end of sin
if(action=="cos")
{
if(radians.isSelected())
{
if(firsttime==false)
{
buf = Math.cos(Double.parseDouble(editor.getText()));
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "cos";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = Math.sin(Double.parseDouble(editor.getText()));
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "cos";
}
}
}
else
{
if(firsttime==false)
{
double rad=Math.toRadians(Double.parseDouble(editor.getText()));
buf = Math.cos(rad);
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "cos";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
double rad=Math.toRadians(Double.parseDouble(editor.getText()));
buf = Math.cos(rad);
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "cos";
}
}
}
}// end of cos
if(action=="tan")
{
if(radians.isSelected())
{
if(firsttime==false)
{
buf = Math.tan(Double.parseDouble(editor.getText()));
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "tan";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
buf = Double.parseDouble(editor.getText());
buf = Math.tan(Double.parseDouble(editor.getText()));
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "tan";
}
}
}
else
{
if(firsttime==false)
{
double rad=Math.toRadians(Double.parseDouble(editor.getText()));
buf = Math.tan(rad);
editor.setText(Double.toString(buf));
opclicked=true;
last_op = "tan";
}
else
{
if(editor.getText()=="")
JOptionPane.showMessageDialog(frame,"Enter input pls...","Input Missing",JOptionPane.ERROR_MESSAGE);
else
{
double rad=Math.toRadians(Double.parseDouble(editor.getText()));
buf = Math.tan(rad);
editor.setText(Double.toString(buf));
firsttime = false;
opclicked=true;
last_op = "tan";
}
}
}
}// end of tan
}
}
}
Comments are welcomed....(Although care has been taken it may contain logical errors or bugs, because I did it in few hours....)
hi .... i am baran , please tell me how to create a jar file for a particular java program ... the above pgm shows ur hardwork ......
ReplyDeleteHi Baran....thanks for u comments....
ReplyDeleteRegarding the JAR file creation, the following link can help you...
http://www.cs.princeton.edu/introcs/85application/jar/jar.html
Hope you like my works and this blog....
hi... now, i find that but it shows some warning error after finishing export operation ..... ( i create a jar file using export->runnable jar file option ) ...please help me , why that warning error come ... ( but it run properly )... u interact me to do something ...... i wish u to do some more in this topic ....
ReplyDeleter u there ?
ReplyDeletethank u very much ,it is very useful for me ... i learn more about jar file creation ......
ReplyDelete